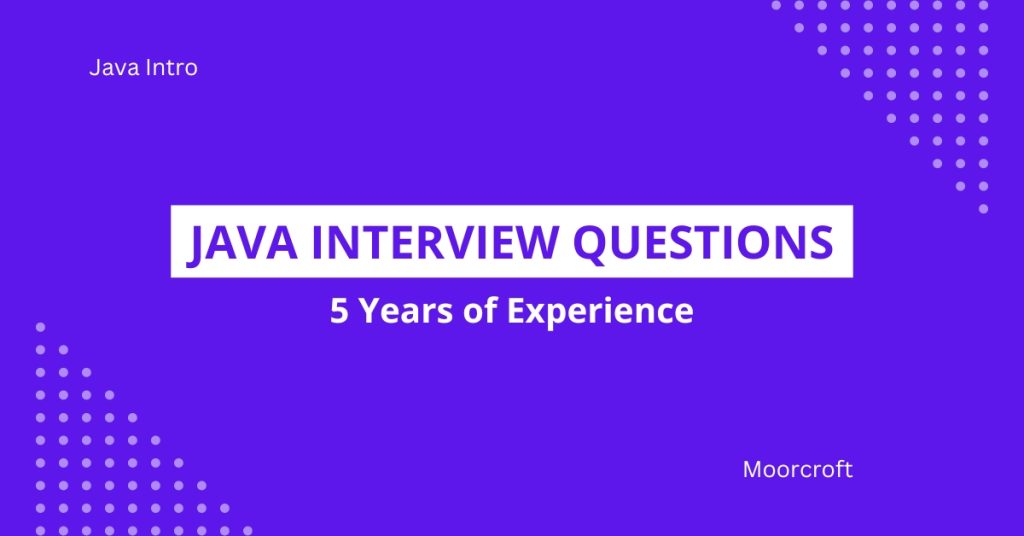
In the ever-evolving field of Java development, experienced professionals often face challenging questions that test not only their knowledge of the language but also their problem-solving skills and experience. Whether you're preparing for a job interview or simply looking to brush up on your skills, this comprehensive guide will help you navigate through the key Java interview questions for professionals with five years of experience.
1. Introduction to Java Interview Questions for Experienced Developers
As a Java developer with five years of experience, you’re expected to have a deep understanding of core Java concepts, advanced features, and practical problem-solving abilities. This guide aims to provide you with a robust set of questions that reflect real-world scenarios and technical challenges you might encounter during interviews. We’ll cover a wide range of topics, including object-oriented programming, concurrency, design patterns, and performance optimization.
2. Core Java Concepts
2.1. What is the difference between HashMap
and Hashtable
?
Answer:
HashMap
is not synchronized and allows one null key and multiple null values. It is preferred for non-thread-safe operations due to better performance.Hashtable
is synchronized, meaning it is thread-safe but may have a performance overhead due to synchronization. It does not allow null keys or values.
2.2. Explain the concept of Java Memory Model
(JMM).
Answer: The Java Memory Model defines how threads interact through memory and what behaviors are allowed in concurrent executions. It ensures visibility of variables and proper ordering of reads and writes to variables across threads.
2.3. What is the purpose of transient
keyword in Java?
Answer: The transient
keyword prevents the serialization of certain fields in an object. When an object is serialized, the transient fields are not included in the serialized representation, thus providing a way to exclude sensitive or non-essential data.
3. Object-Oriented Programming (OOP) Principles
3.1. How does Java achieve polymorphism?
Answer: Java achieves polymorphism through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism). Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. Method overloading allows multiple methods with the same name but different parameters.
3.2. What are abstract classes and interfaces? How do they differ?
Answer:
- Abstract Classes: Can have both abstract methods (without implementations) and concrete methods (with implementations). They can also have instance variables and constructors.
- Interfaces: Can only have abstract methods until Java 8 (since Java 8, they can also have default and static methods). Interfaces cannot have instance variables or constructors.
4. Advanced Java Topics
4.1. Explain the concept of Java Streams
and its advantages.
Answer: Java Streams provide a functional approach to processing sequences of elements, such as collections. They support operations like filtering, mapping, and reducing in a declarative manner. Streams offer advantages such as parallel processing, lazy evaluation, and cleaner code.
4.2. What are lambda expressions
and where are they used?
Answer: Lambda expressions provide a concise way to represent anonymous functions or instances of single-method interfaces (functional interfaces). They are used primarily in functional programming and can simplify the syntax of passing behavior as parameters.
5. Concurrency and Multithreading
5.1. What is the difference between synchronized
block and Lock
interface in Java?
Answer:
- Synchronized Block: Provides a simple way to ensure that only one thread can access a block of code at a time. It is less flexible but easy to use.
- Lock Interface: Part of the
java.util.concurrent.locks
package, it provides more sophisticated locking mechanisms like timed and interruptible locks, and can be used to achieve finer-grained control over concurrency.
5.2. How do you handle thread safety in Java?
Answer: Thread safety can be achieved through synchronization mechanisms such as synchronized
blocks, locks (ReentrantLock
, ReadWriteLock
), atomic variables (AtomicInteger
, AtomicReference
), and concurrent collections (ConcurrentHashMap
, CopyOnWriteArrayList
).
6. Design Patterns
6.1. Describe the Singleton Design Pattern and provide a sample implementation.
Answer: The Singleton Design Pattern ensures that a class has only one instance and provides a global point of access to that instance.
Sample Implementation:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
6.2. What is the Factory Method Pattern?
Answer: The Factory Method Pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. It promotes loose coupling by delegating the instantiation logic to subclasses.
7. Performance and Optimization
7.1. How can you improve the performance of a Java application?
Answer: Performance improvements can be achieved by optimizing algorithms, reducing object creation, using efficient data structures, minimizing synchronization, and employing profiling tools to identify bottlenecks.
7.2. What are the differences between String
, StringBuilder
, and StringBuffer
?
Answer:
- String: Immutable, meaning once created, its value cannot be changed.
- StringBuilder: Mutable, provides a way to create and manipulate strings efficiently without creating new objects. Not synchronized.
- StringBuffer: Mutable and synchronized, used in multi-threaded environments where thread safety is a concern.
8. Java 8 Features
8.1. What are default
methods in interfaces?
Answer: Default methods in interfaces allow you to add new methods to interfaces with default implementations. This feature helps in extending interfaces without breaking the existing implementations.
8.2. Explain the use of Optional
in Java.
Answer: Optional
is a container object which may or may not contain a value. It is used to avoid null checks and NullPointerException
by providing methods to handle the presence or absence of a value in a more functional manner.
9. Exception Handling
9.1. What is the difference between checked and unchecked exceptions?
Answer:
- Checked Exceptions: Must be either caught or declared in the method’s
throws
clause. Examples includeIOException
andSQLException
. - Unchecked Exceptions: Do not need to be explicitly caught or declared. Examples include
RuntimeException
,NullPointerException
, andArrayIndexOutOfBoundsException
.
9.2. How do you create a custom exception in Java?
Answer: To create a custom exception, you need to extend the Exception
or RuntimeException
class and provide a constructor to pass a custom message or cause.
Sample Implementation:
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
10. Java Frameworks and Libraries
10.1. What is Spring Framework and how does it work?
Answer: Spring Framework is a comprehensive framework for enterprise Java development. It provides support for dependency injection, aspect-oriented programming, and transaction management. It helps in building scalable and maintainable applications by promoting loose coupling and separation of concerns.
10.2. What is Hibernate and how is it used for ORM?
Answer: Hibernate is an Object-Relational Mapping (ORM) framework that simplifies database interactions in Java. It maps Java classes to database tables, handles data retrieval and manipulation, and manages relationships between entities.
11. Java Collections Framework
11.1. Explain the differences between ArrayList
and LinkedList
.
Answer:
- ArrayList: Backed by an array, provides fast random access and is better for scenarios where frequent access is required.
- LinkedList: Doubly-linked list implementation, provides efficient insertions and deletions but slower random access compared to
ArrayList
.
11.2. How does ConcurrentHashMap
work?
Answer: ConcurrentHashMap
is a thread-safe implementation of Map
that allows concurrent read and write operations. It uses a segmented locking mechanism to provide high concurrency and reduce contention.
12. Mock MCQs for Java Interview Preparation
To help you prepare effectively, here are 10 multiple-choice questions based on the topics discussed:
- Which of the following classes is synchronized?
- a)
HashMap
- b)
TreeMap
- c)
Hashtable
- d)
LinkedHashMap
- a)
- What is the output of the following code snippet?
String str = "Java";
str.toLowerCase();
System.out.println(str);
- a)
java
- b)
JAVA
- c)
Java
- d)
null
- Which keyword is used to prevent the serialization of an instance variable?
- a)
volatile
- b)
transient
- c)
static
- d)
final
- a)
- Which of the following is true about Java 8 lambda expressions?
- a) They can have multiple statements.
- b) They cannot access local variables.
- c) They can only be used with interfaces.
- d) They do not support type inference.
- What is the default access modifier for a class member?
- a)
public
- b)
private
- c)
protected
- d)
default
- a)
- Which design pattern provides a global point of access to an instance?
- a) Singleton
- b) Factory
- c) Observer
- d) Builder
- What is the primary use of
java.util.concurrent
package?- a) To provide utility classes for data structures.
- b) To handle I/O operations.
- c) To support multi-threading and concurrency.
- d) To manage serialization.
- Which method in
Optional
can be used to get the value if present or provide a default?- a)
get()
- b)
orElse()
- c)
ifPresent()
- d)
isPresent()
- a)
- Which class is not part of the Java Collections Framework?
- a)
ArrayList
- b)
HashSet
- c)
LinkedList
- d)
LinkedHashMap
- a)
- In which scenario would you prefer using
StringBuilder
overString
?- a) When immutability is required.
- b) For efficient concatenation of strings.
- c) For thread-safe operations.
- d) For defining constant strings.
13. Conclusion
Preparing for a Java interview with five years of experience involves not only understanding core concepts and advanced features but also being able to demonstrate practical problem-solving skills. This guide provides a thorough set of questions and answers to help you review and refine your knowledge. By mastering these topics, you'll be well-equipped to tackle challenging interview questions and advance in your Java development career.
Comments (0)