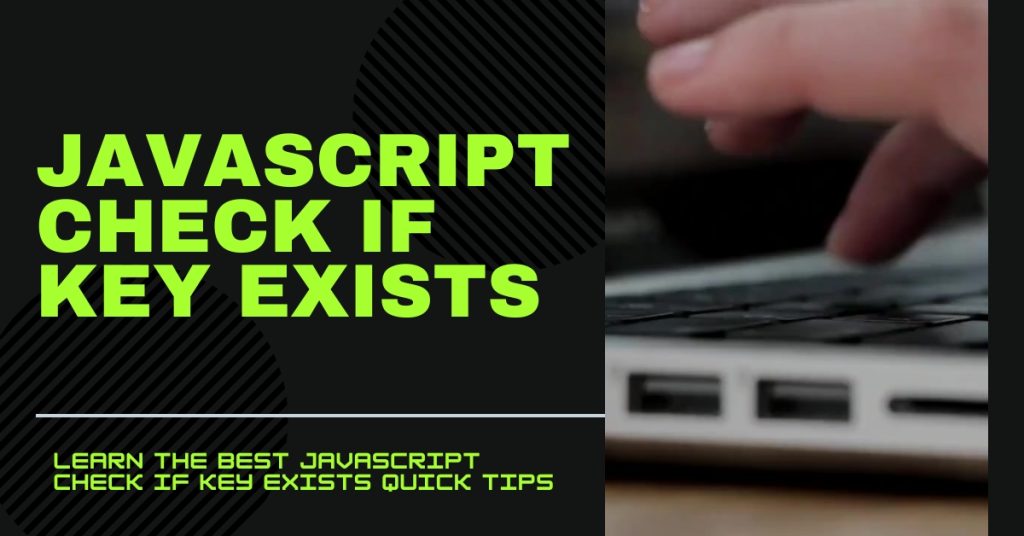
Checking Key Existence in JavaScript A Comprehensive Guide
In JavaScript, verifying if a key exists in an object or array is crucial for ensuring smooth code execution. Multiple methods are available to accomplish this task.
Key Takeaways
in
Operator andhasOwnProperty()
Method: These are commonly used to check for key existence in JavaScript objects and arrays.in
Operator: Simple and reliable for essential existence checks, it also includes inherited properties.hasOwnProperty()
Method: Excludes inherited properties, focusing solely on the object's own keys.- Undefined Values: Checking for undefined values is not a reliable method for determining key existence.
- Performance Considerations: When working with large datasets, consider the performance implications of your chosen method.
Using the in
Operator
The in
operator provides a straightforward way to check if a key exists in an object or array, including its prototype chain.
codeconst person = {
name: "John Doe",
age: 30,
city: "New York"
};
console.log('name' in person); // Outputs true
console.log('occupation' in person); // Outputs false
Benefits of Using the in
Operator
- Clarity and Ease: Simple syntax for checking key existence.
- Versatility: Works with both objects and arrays.
- Prototype Chain: Checks the entire prototype chain for key existence.
Comparing in
Operator and hasOwnProperty()
Method | Objects | Arrays | Checks Prototype Chain |
---|---|---|---|
in Operator | ✅ | ✅ | ✅ |
hasOwnProperty() | ✅ | ❌ | ❌ |
Using the hasOwnProperty()
Method
The hasOwnProperty()
method checks if an object has a specific key as its own property, excluding inherited keys.
codelet person = {
name: 'John',
age: 25,
};
if (person.hasOwnProperty('name')) {
console.log('Name key exists in the object!');
} else {
console.log('Name key does not exist in the object.');
}
Handling Undefined Values
Using the in
operator or hasOwnProperty()
method is more reliable than checking for undefined values to determine key existence.
codeconst person = {
name: 'John Doe',
age: 30,
// address: undefined,
};
console.log('address' in person); // Output: true
console.log(person.hasOwnProperty('address')); // Output: true
Performance Comparison
in
Operator: May be slower due to searching the prototype chain.hasOwnProperty()
Method: Potentially faster by skipping the prototype chain search.
Alternative Approaches
The optional chaining operator (?.
) is another method for safely accessing nested keys without throwing errors.
javascript codevar city = person?.address?.city;
Best Practices for Key Existence Checking
- Use the
in
Operator orhasOwnProperty()
Method: Avoid checking for undefined values. - Consider Performance: Choose the method based on your dataset size.
- Mind the Syntax: Use the appropriate method for your specific needs.
Common Pitfalls to Avoid
- Relying on Undefined Checks: This does not reliably indicate key existence.
- Using the Wrong Method: Choose the right method for objects or arrays to avoid errors.
Conclusion
Selecting the right method to check key existence in JavaScript—either the in
operator or hasOwnProperty()
method—ensures your code is reliable and efficient. Avoid pitfalls like relying on undefined checks, and follow best practices for optimal results.
Comments (0)